程序员面试题精选100题(31)-从尾到头输出链表
字号:小|大
2019-09-22 FW.5VV.CN范文网
程序员面试题精选100题(31)-从尾到头输出链表
题目:输入一个链表的头结点,从尾到头反过来输出每个结点的值。链表结点定义如下:
struct ListNode
{
int m_nKey;
ListNode* m_pNext;
};
方法一:
看到这道题后,第一反应是从头到尾输出比较简单。于是很自然地想到把链表中链接结点的指针反转过来,改变链表的方向。然后就可以从头到尾输出了。
void PrintListReversingly(ListNode* pHead)
{
ListNode* pReverseHead=NULL;
ListNode* cursor=pHead;
//翻转链表
while(cursor!=NULL)
{
ListNode* next=cursor->next;
if(NULL==pReverseHead){
cursor->m_pNext=NULL;
}else{
cursor->m_pNext=pReverseHead;
}
pReverseHead=cursor;
cursor=next;
}
cursor=pReverseHead;//指向翻转后的链表的头
while(cursor!=NULL)
{
printf("%d ",cursor->m_nKey);
cursor=cursor->m_pNext;
}
}
方法二:
接下来的想法是从头到尾遍历链表,每经过一个结点的时候,把该结点放到一个栈中。当遍历完整个链表后,再从栈顶开始输出结点的值,此时输出的结点的顺序已经反转过来了。该方法需要维护一个额外的栈,实现起来比较麻烦。
void PrintListReversingly_Iteratively(ListNode* pHead)
{
std::stack<ListNode*> nodes;
ListNode* pNode=pHead;
while(pNode!=NULL)
{
nodes.push(pNode);
pNode=pNode->m_pNext;
}
while(!nodes.empty())
{
pNode=nodes.top();
printf("%d\t",pNode->m_nKey);
nodes.pop();
}
}
方法三:
既然想到了栈来实现这个函数,而递归本质上就是一个栈结构。于是很自然的又想到了用递归来实现。要实现反过来输出链表,我们每访问到一个结点的时候,先递归输出它后面的结点,再输出该结点自身,这样链表的输出结果就反过来了。
基于这样的思路,不难写出如下代码:
///////////////////////////////////////////////////////////////////////
// Print a list from end to beginning
// Input: pListHead - the head of list
///////////////////////////////////////////////////////////////////////
void PrintListReversely(ListNode* pListHead)
{
if(pListHead != NULL)
{
// Print the next node first
if (pListHead->m_pNext != NULL)
{
PrintListReversely(pListHead->m_pNext);
}
// Print this node
printf("%d", pListHead->m_nKey);
}
}
题目:输入一个链表的头结点,从尾到头反过来输出每个结点的值。链表结点定义如下:
struct ListNode
{
int m_nKey;
ListNode* m_pNext;
};
方法一:
看到这道题后,第一反应是从头到尾输出比较简单。于是很自然地想到把链表中链接结点的指针反转过来,改变链表的方向。然后就可以从头到尾输出了。
void PrintListReversingly(ListNode* pHead)
{
ListNode* pReverseHead=NULL;
ListNode* cursor=pHead;
//翻转链表
while(cursor!=NULL)
{
ListNode* next=cursor->next;
if(NULL==pReverseHead){
cursor->m_pNext=NULL;
}else{
cursor->m_pNext=pReverseHead;
}
pReverseHead=cursor;
cursor=next;
}
cursor=pReverseHead;//指向翻转后的链表的头
while(cursor!=NULL)
{
printf("%d ",cursor->m_nKey);
cursor=cursor->m_pNext;
}
}
方法二:
接下来的想法是从头到尾遍历链表,每经过一个结点的时候,把该结点放到一个栈中。当遍历完整个链表后,再从栈顶开始输出结点的值,此时输出的结点的顺序已经反转过来了。该方法需要维护一个额外的栈,实现起来比较麻烦。
void PrintListReversingly_Iteratively(ListNode* pHead)
{
std::stack<ListNode*> nodes;
ListNode* pNode=pHead;
while(pNode!=NULL)
{
nodes.push(pNode);
pNode=pNode->m_pNext;
}
while(!nodes.empty())
{
pNode=nodes.top();
printf("%d\t",pNode->m_nKey);
nodes.pop();
}
}
方法三:
既然想到了栈来实现这个函数,而递归本质上就是一个栈结构。于是很自然的又想到了用递归来实现。要实现反过来输出链表,我们每访问到一个结点的时候,先递归输出它后面的结点,再输出该结点自身,这样链表的输出结果就反过来了。
基于这样的思路,不难写出如下代码:
///////////////////////////////////////////////////////////////////////
// Print a list from end to beginning
// Input: pListHead - the head of list
///////////////////////////////////////////////////////////////////////
void PrintListReversely(ListNode* pListHead)
{
if(pListHead != NULL)
{
// Print the next node first
if (pListHead->m_pNext != NULL)
{
PrintListReversely(pListHead->m_pNext);
}
// Print this node
printf("%d", pListHead->m_nKey);
}
}
相关文章
- 程序员面试题精选100题(03)-第一个只出现一次的字符[算法]
- 程序员面试题精选100题(21)-左旋转字符串[算法]
- 程序员面试题精选100题(24)-栈的push、pop序列[数据结构]
- 程序员面试题精选100题(26)-和为n连续正数序列[算法]
- 程序员面试题精选100题(32)-不能被继承的类[C/C++/C#]
- 程序员面试题精选100题(46)-对称子字符串的最大长度[算法]
- 程序员面试题精选100题(04)-把字符串转换成整数
- 程序员面试题精选100题(10)-排序数组中和为给定值的两个数字[算法]
- 程序员面试题精选100题(16)-O(logn)求Fibonacci数列[算法]
- 程序员面试题精选100题(17)-把字符串转换成整数[算法]
热门推荐
- 程序员面试题精选100题(03)-第一个只出现一次的字符[算法]
- 程序员面试题精选100题(21)-左旋转字符串[算法]
- 程序员面试题精选100题(24)-栈的push、pop序列[数据结构]
- 程序员面试题精选100题(26)-和为n连续正数序列[算法]
- 程序员面试题精选100题(32)-不能被继承的类[C/C++/C#]
- 程序员面试题精选100题(46)-对称子字符串的最大长度[算法]
- 程序员面试题精选100题(04)-把字符串转换成整数
- 程序员面试题精选100题(10)-排序数组中和为给定值的两个数字[算法]
- 程序员面试题精选100题(16)-O(logn)求Fibonacci数列[算法]
- 程序员面试题精选100题(17)-把字符串转换成整数[算法]
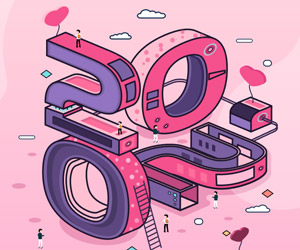